Simplifying the MCP23017 - written by NiXijav on 2016-03-01
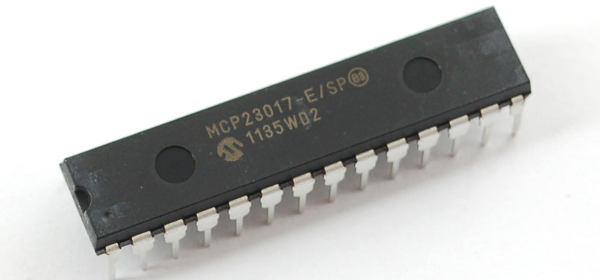
Library for the Raspberry Pi written in Python that simplifies the use of the MCP23017, a 16 bit GPIO expander
The MCP23017 is a very useful IC. It provides 16 GPIOs, and it's ideal for expanding the ports of a Raspberry Pi. It even includes pullup resistors for each pin, and all of this using i2c.
You can find the datasheet for this chip here
But all this benefits come at a price. It is not easy to use, or at least, not easy to use initially. The MCP23017 operates entirely based on registers (Page 9 of the datasheet), and depending on which register you are writing to, you'll be able to change the direction of the pins, state, pullups... Also, GPIOs are divided into two different banks, and they are independent from each other.
To simplify this boring register thing, I created a library, in Python, for the Raspberry Pi. Commands are almost the same as the RPi.GPIO ones.
Here is the pinout the library uses:
A very simple code example:
```import mcp23017_lib as MCP
MCP.start(0x26)
MCP.setup(2, MCP.IN, MCP.PUHIGH)
MCP.setup(1, MCP.OUT)
while 1:
if(MCP.input(2)):
MCP.write(1, MCP.HIGH)
else:
MCP.write(1, MCP.LOW)
```
This example will set pin #2 as an input, with the pullup resistor enabled and #1 as an output. When the input goes high, pin #1 will also go high.
You can find the library here. It is licensed under the GPLv3.
If you found this library useful, and/or have suggestions, please let me know in a comment :3
Comments (2)
Hello Ingmar, I just noticed there's an error on the sample code, and 'read' should be replaced by 'input', so MCP.read(2) becomes MCP.input(2). Sorry for the delay, and best of luck!
Hi NiXijav, I tried to run you example code but I get the following error: pi@robi:~/mcp $ sudo python mcp_test.py Traceback (most recent call last): File "mcp_test.py", line 9, in if(MCP.read(2)): AttributeError: 'module' object has no attribute 'read' pi@robi:~/mcp $ I forked you GitHub project and tried to use a different MCP23017 chip. You see a picture on my GitHub repository. https://github.com/custom-build-robots/mcp23017_lib Could you help me with the error please? I think the error has nothing to do with the used chip so far. Thank you very much, Ingmar
Leave a comment